- Mistral integration
- Edge or MCU application of the model
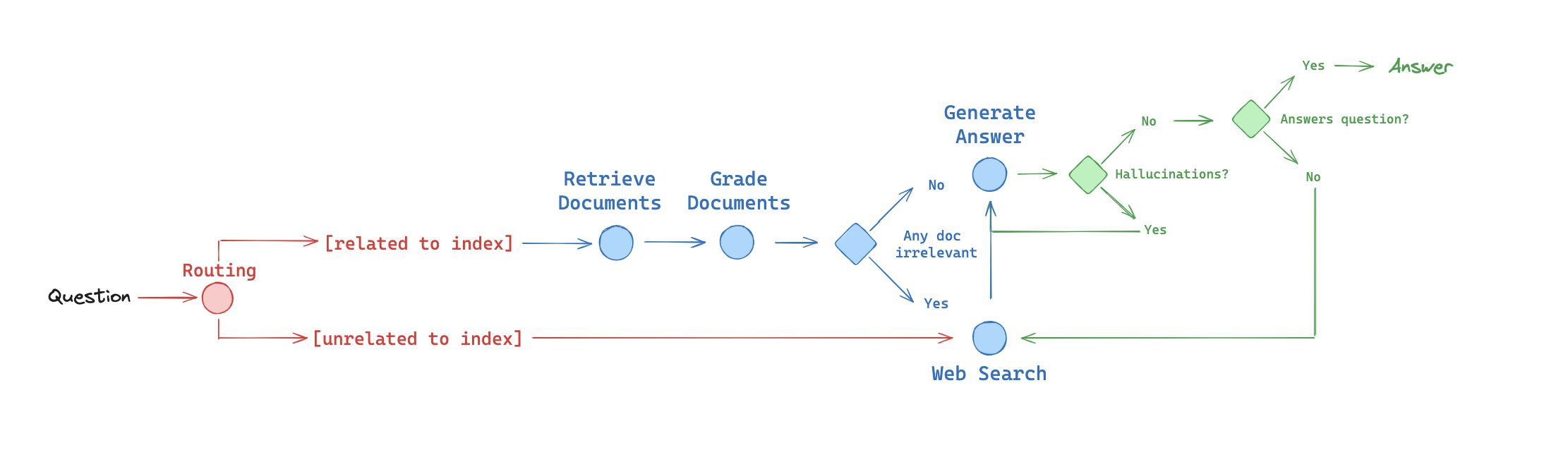
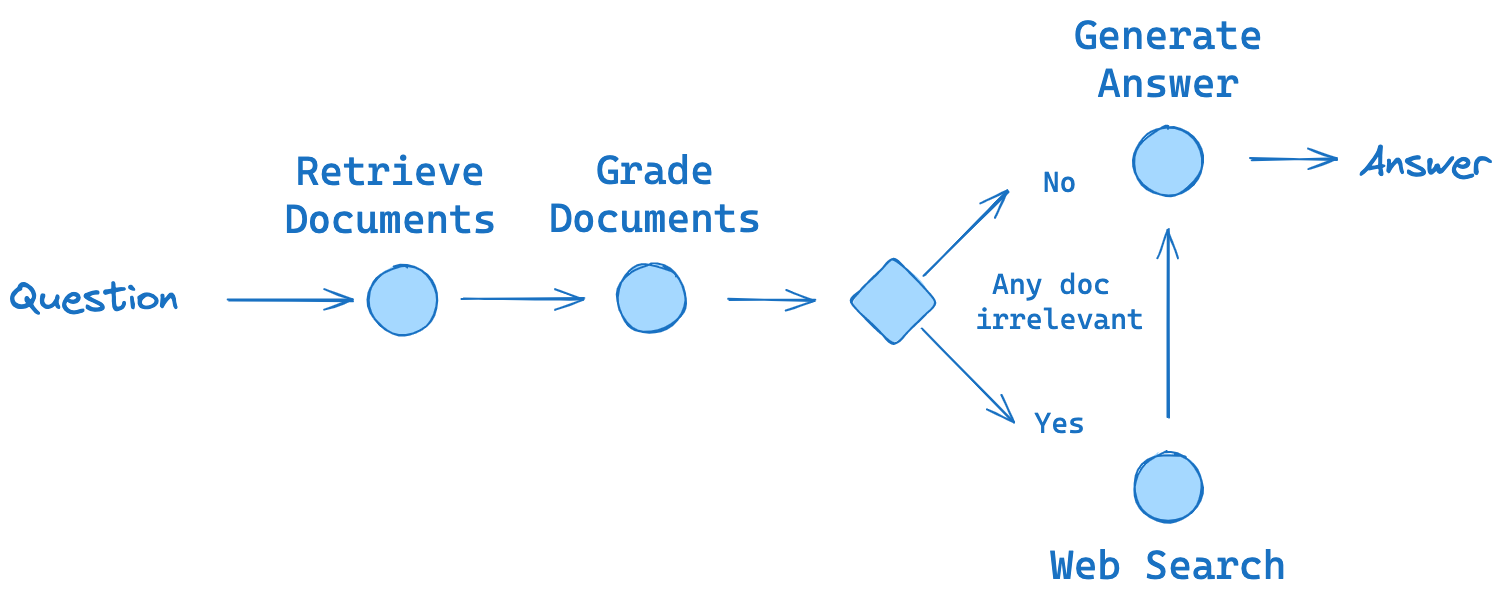
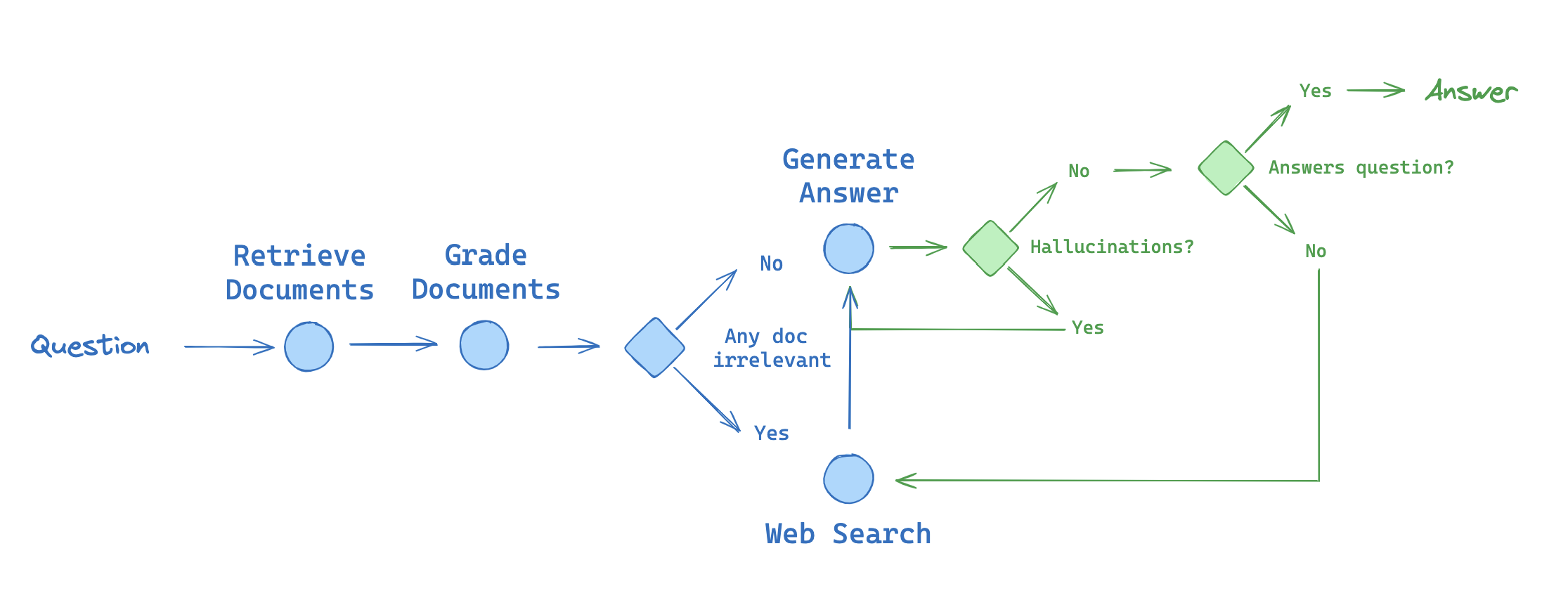
</div></p></details>
Mistral integration
Building an edge model using Mistral 7B involves several steps, from setting up your environment to deploying the model on an edge device. Below is a high-level guide on how to go about it:
1. Setting Up Your Environment
First, you need to set up your environment. This involves installing the necessary libraries and dependencies.
Prerequisites
- Python 3.7+
- PyTorch
- Transformers library from Hugging Face
- Other necessary libraries
Example: Setting Up Environment
# Create a virtual environment
python3 -m venv mistral-env
source mistral-env/bin/activate
# Upgrade pip
pip install --upgrade pip
# Install PyTorch (select the right version based on your CUDA version)
pip install torch torchvision torchaudio
# Install Hugging Face Transformers and other libraries
pip install transformers datasets
2. Download the Model
You can download the Mistral 7B model from Hugging Face’s model hub.
Example: Downloading the Model
from transformers import AutoModelForCausalLM, AutoTokenizer
# Load the model and tokenizer
model_name = "mistralai/Mistral-7B-v0.1"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModelForCausalLM.from_pretrained(model_name)
3. Model Inference
You can now perform inference using the loaded model. This involves tokenizing the input text, generating a response, and decoding the output.
Example: Running Inference
# Sample input text
input_text = "Once upon a time"
# Tokenize the input text
inputs = tokenizer(input_text, return_tensors="pt")
# Generate a response
outputs = model.generate(inputs.input_ids, max_length=50, num_return_sequences=1)
# Decode the generated text
generated_text = tokenizer.decode(outputs[0], skip_special_tokens=True)
print(generated_text)
4. Optimizing the Model for Edge Deployment
Running large models like Mistral 7B on edge devices can be challenging due to resource constraints. You can optimize the model using techniques like quantization and pruning.
Quantization
Quantization reduces the model size by converting the weights from 32-bit floating point to 8-bit integers.
Example: Quantizing the Model
import torch.quantization
# Quantize the model
model.eval()
quantized_model = torch.quantization.quantize_dynamic(
model, {torch.nn.Linear}, dtype=torch.qint8
)
5. Deploying on Edge Device
Deploy the quantized model on an edge device. This may involve transferring the model to the device and writing a script to load and run the model.
Example: Deploying on Raspberry Pi
- Transfer Model Files
- Transfer the model files to your Raspberry Pi using
scp
or a similar method.
- Transfer the model files to your Raspberry Pi using
-
Install Dependencies on Raspberry Pi
# SSH into your Raspberry Pi ssh pi@your_pi_ip_address # Install dependencies sudo apt-get update sudo apt-get install python3-pip pip3 install torch transformers
-
Run the Inference Script
# Save the following script as run_model.py on your Raspberry Pi from transformers import AutoModelForCausalLM, AutoTokenizer # Load the model and tokenizer model_name = "path_to_quantized_model" # Change this to the path where your quantized model is saved tokenizer = AutoTokenizer.from_pretrained(model_name) model = torch.load(model_name) # Sample input text input_text = "Once upon a time" # Tokenize the input text inputs = tokenizer(input_text, return_tensors="pt") # Generate a response outputs = model.generate(inputs.input_ids, max_length=50, num_return_sequences=1) # Decode the generated text generated_text = tokenizer.decode(outputs[0], skip_special_tokens=True) print(generated_text)
# Run the script python3 run_model.py
6. Monitoring and Maintenance
Ensure you have monitoring in place to track the performance and resource utilization of the model on the edge device. Regular maintenance and updates may be required to keep the model performing optimally.
Resources
I hope I can successfully deploy a Mistral 7B model on an edge device, ensuring efficient and optimized performance for your specific use case.
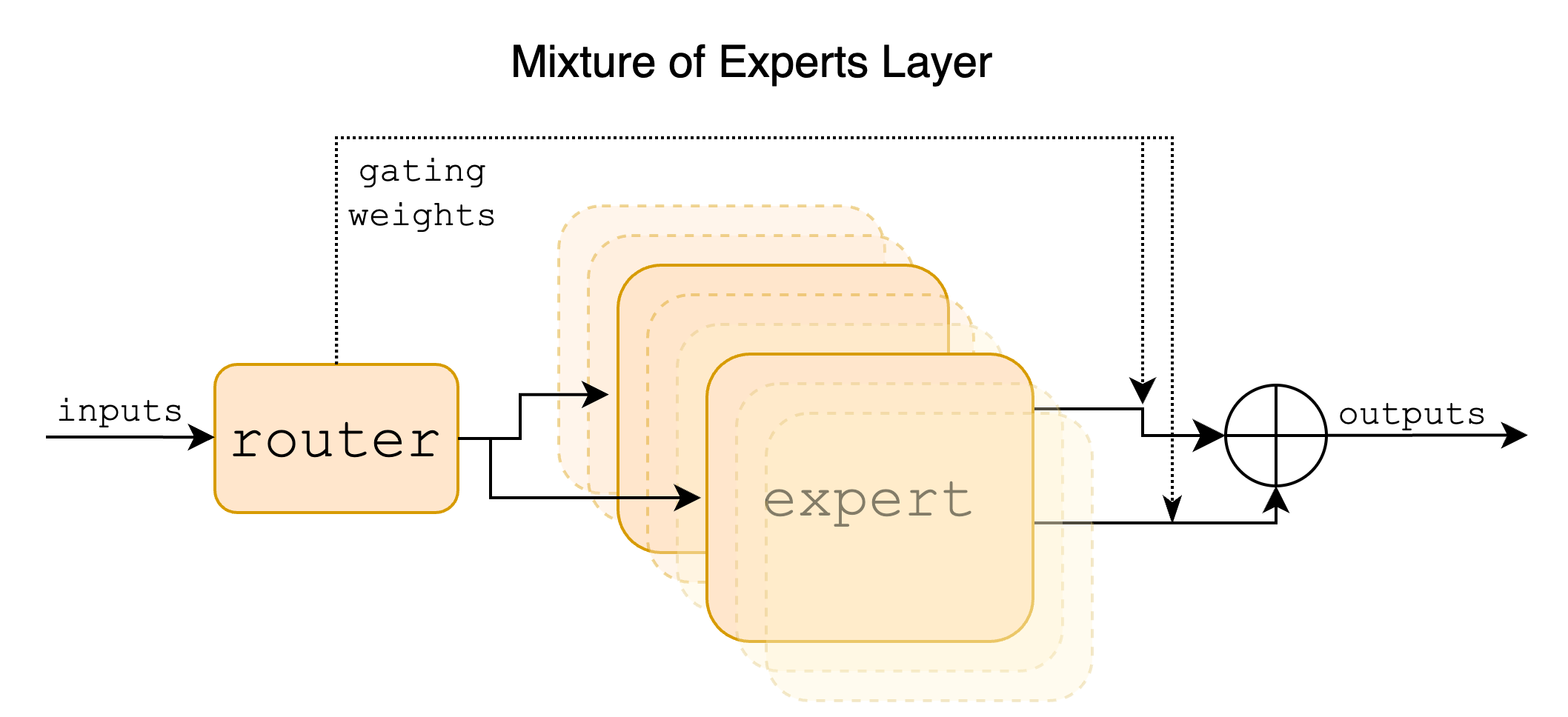
Edge or MCU application of the model
Deploying a large language model like Mistral 7B directly on an MCU (Microcontroller Unit) is a challenging task due to the limited computational power and memory of such devices. However, deploying a simplified, optimized version or using other frameworks can make it feasible. Here’s a more detailed approach focusing on setting up on edge devices and using frameworks beyond API calls to Mistral.
1. Optimizing the Model for Edge Deployment
To deploy on an MCU or similar constrained device, you need to optimize the model extensively. This includes:
- Quantization: Reducing the precision of the model weights.
- Pruning: Removing parts of the model that contribute less to the output.
- Model Distillation: Training a smaller model to replicate the behavior of a larger model.
2. Using TinyML Frameworks
Frameworks like TensorFlow Lite for Microcontrollers and ONNX Runtime can help in running models on MCUs.
3. Example: Using TensorFlow Lite for Microcontrollers
Step 1: Convert Model to TensorFlow Lite
First, convert the trained model to TensorFlow Lite format.
import tensorflow as tf
from transformers import TFAutoModelForCausalLM
# Load the pre-trained model
model_name = "mistralai/Mistral-7B-v0.1"
model = TFAutoModelForCausalLM.from_pretrained(model_name, from_pt=True)
# Convert to TensorFlow Lite
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
# Save the model
with open("mistral_model.tflite", "wb") as f:
f.write(tflite_model)
Step 2: Optimize the TensorFlow Lite Model
Optimize the model for edge devices.
# Use TFLite Converter for optimization
converter.optimizations = [tf.lite.Optimize.DEFAULT]
tflite_quantized_model = converter.convert()
# Save the quantized model
with open("mistral_model_quantized.tflite", "wb") as f:
f.write(tflite_quantized_model)
Step 3: Deploying on an MCU
Use TensorFlow Lite for Microcontrollers to run the model.
-
Install TensorFlow Lite for Microcontrollers
Follow the installation guide for TensorFlow Lite for Microcontrollers specific to your MCU.
-
Load and Run the Model
Write the necessary code to load and run the TensorFlow Lite model on your MCU.
// Include the TensorFlow Lite header files #include "tensorflow/lite/micro/all_ops_resolver.h" #include "tensorflow/lite/micro/micro_interpreter.h" #include "tensorflow/lite/micro/micro_mutable_op_resolver.h" #include "tensorflow/lite/schema/schema_generated.h" #include "tensorflow/lite/version.h" // Load the model from the file const tflite::Model* model = tflite::GetModel(mistral_model_quantized_tflite); if (model->version() != TFLITE_SCHEMA_VERSION) { printf("Model provided is schema version %d not equal to supported version %d.", model->version(), TFLITE_SCHEMA_VERSION); return 1; } // Set up the interpreter static tflite::MicroMutableOpResolver<10> micro_op_resolver; tflite::MicroInterpreter interpreter( model, micro_op_resolver, tensor_arena, tensor_arena_size, error_reporter); // Allocate memory from the tensor_arena for the model's tensors. TfLiteStatus allocate_status = interpreter.AllocateTensors(); if (allocate_status != kTfLiteOk) { printf("AllocateTensors() failed"); return 1; } // Obtain pointers to the model's input and output tensors. TfLiteTensor* input = interpreter.input(0); TfLiteTensor* output = interpreter.output(0); // Fill the input tensor with your data. // TODO: Add code to populate input data // Run inference TfLiteStatus invoke_status = interpreter.Invoke(); if (invoke_status != kTfLiteOk) { printf("Invoke failed"); return 1; } // Process the output data. // TODO: Add code to handle the output data
4. Using ONNX Runtime
Another option is using ONNX Runtime, which supports deployment on edge devices and can be used with optimized models.
Step 1: Convert the Model to ONNX
Convert your model to ONNX format.
import torch
from transformers import AutoModelForCausalLM
# Load the pre-trained model
model_name = "mistralai/Mistral-7B-v0.1"
model = AutoModelForCausalLM.from_pretrained(model_name)
# Create dummy input for tracing
dummy_input = torch.randint(0, 50256, (1, 1))
# Export the model
torch.onnx.export(model, dummy_input, "mistral_model.onnx", opset_version=11)
Step 2: Optimize the ONNX Model
Use ONNX tools to optimize the model for edge deployment.
python -m onnxruntime.transformers.optimizer --input mistral_model.onnx --output mistral_model_optimized.onnx
Step 3: Deploying on an Edge Device
Use ONNX Runtime to run the model on an edge device.
import onnxruntime as ort
import numpy as np
# Load the ONNX model
session = ort.InferenceSession("mistral_model_optimized.onnx")
# Prepare input data
input_data = np.random.randint(0, 50256, (1, 1)).astype(np.int64)
# Run inference
outputs = session.run(None, {"input_ids": input_data})
print(outputs)
Conclusion
Deploying a large model like Mistral 7B on an MCU is challenging and typically requires significant optimization and use of specialized frameworks. By converting the model to formats supported by TensorFlow Lite or ONNX Runtime, and optimizing it through quantization and other techniques, you can run the model on constrained devices. This allows you to leverage the power of large language models in edge applications, enabling local processing with reduced latency and dependence on network connectivity.
The following wiki, pages and posts are tagged with
Title | Type | Excerpt |
---|---|---|
locally serving llm chatbots | post | Tue, Jun 11, 24, using langchain production ready llmrag |